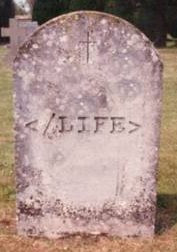
Want to see something that will blow your mind? Something that will shoot your brain out the back of your skull, through the window, and onto your neighbor's freshly waxed Pinto?
<?xml version="1.0" encoding="UTF-8"?>
<?xml-stylesheet type="text/xsl" href="layout/frontpage.xsl"?>
<page lang="en_us">
<frontpage/>
</page>
This is the source code for a website's entire home page (which is that of the upcoming computer game Starcraft 2 by Blizzard Entertainment).
I could be in the minority, but I've never seen XSLT used to transform XML documents into web pages...and I've never realized how powerful it can be. After exploring the source code of the website, here are some advantages and disadvantages of this technique that I've discovered:
Advantage - Separation of concerns
Using XSLT, you can more effectively separate the content of your website from the presentation. The Starcraft 2 website has *all* of its text separated out into XML files (pretty cool!). For example, you can find all the text on the Terran page, in a file devoted solely to storing Terran-related text (terran.xml).
Advantage - i18n
By separating the content from the presentation, another benefit is that it makes it easy to internationalize the website. This is what Blizzard has done. The XML files used to store text are located in their own directory, named according to the language of the text (such as "fr_fr" for French). The language of the guy viewing the website is stored in the "lang" attribute of "page" tag of each individual web page (see the above code snippet). This attribute is used to determine which directory to retrieve the text from.
Also, you can do things that you would normally think could only be done using server-side languages:
Advantage - Access to special functions
Since XSLT uses the XPath language, you get to use all the special functions that XPath comes with. Tasks like string manipulation, date/time formatting, rounding numbers--all things that one might typically associate only with server-side languages, can all be done with XPath. For example, the following code, taken from a shared file called includes.xsl, uses the "concat" function to build a URI that points to a language-specific version of an XML file:
<xsl:variable name="terran" select="document(concat('/strings/',$lang,'/terran.xml'))"/>
Advantage - Avoiding code duplication
Just as you might define a function in a server-side script that performs some task, you can define function-like constructs with XSLT that "output" HTML. If you look at movies.xsl (a file used to generate the Movies page) you can see that for each movie listed on the page, a template is called:
<xsl:call-template name="movies-entry">
<xsl:with-param name="title" select="$loc/strs/str[@id='sc2.labels.movies.movie6.title']"/>
<xsl:with-param name="movieid" select="'6'"/>
... more parameters ...
</xsl:call-template>
The template, defined in a shared file called includes.xsl, contains the HTML code used to display the movie:
<xsl:template name="movies-entry">
<xsl:param name="title"/>
<xsl:param name="movieid"/>
... more parameters ...
... HTML/XSL code used to display the movie ...
</xsl:template>
So instead of having to do a lot of copying and pasting, as you would have to do if using plain HTML, the code stays centralized in one place, making bug fixes and other maintenance tasks easier to handle.
Disadvantage - Backward-compatibility
XSLT didn't become a W3C recommendation until 1999, so any browser created before or around that time probably won't have strong support for the language (this includes IE 5 and earlier). So you have to consider the software your audience is likely to be using before building your site. Blizzard probably thought it safe to use this technology, since gamers tend to use the latest and greatest browsers and are therefore unlikely to run into any compatibility issues.
So in conclusion, I've found that using XSLT to build web sites gives you many advantages that you don't get when developing with vanilla HTML. Perhaps I will use it for my next web development project!